To create a coming soon page with HTML , you can use the following template , you can use notepad or any other text/code editor to create this , save this file as index.html and upload it to your host .
<!DOCTYPE html>
<html>
<head>
<title>Coming Soon</title>
</head>
<body>
<h1>Coming soon!</h1>
<p>Thanks for visiting. We're currently working on something new, so please check back in a bit.</p>
</body>
</html>
This template creates a simple page with a heading and a paragraph . You can customize the content to fit your needs. For example you could add a countdown timer or a newsletter sign up form to the page.
Here s an example of how you can create a coming soon page using HTML, with a Countdown Timer , background image and font colors
<!DOCTYPE html>
<html>
<head>
<title>Coming Soon</title>
<style>
body {
background-image: url("background-image.jpg");
background-size: cover;
font-family: Arial, sans-serif;
}
h1 {
color: white;
text-align: center;
font-size: 2em;
margin-top: 50px;
}
p {
color: white;
text-align: center;
font-size: 1.5em;
}
#countdown {
color: white;
font-size: 1.5em;
text-align: center;
margin-top: 20px;
}
</style>
</head>
<body>
<h1>Coming soon!</h1>
<p>Thanks for visiting. We're currently working on something new, and we'll be launching in:</p>
<div id="countdown"></div>
<script>
// Set the date we're counting down to
var countDownDate = new Date("Jan 25, 2024 15:37:25").getTime();
// Update the count down every 1 second
var x = setInterval(function() {
// Get today's date and time
var now = new Date().getTime();
// Find the distance between now and the count down date
var distance = countDownDate - now;
// Time calculations for days, hours, minutes and seconds
var days = Math.floor(distance / (1000 * 60 * 60 * 24));
var hours = Math.floor((distance % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
var minutes = Math.floor((distance % (1000 * 60 * 60)) / (1000 * 60));
var seconds = Math.floor((distance % (1000 * 60)) / 1000);
// Output the result in an element with id="countdown"
document.getElementById("countdown").innerHTML = days + "d " + hours + "h "
+ minutes + "m " + seconds + "s ";
// If the count down is over, write some text
if (distance < 0) {
clearInterval(x);
document.getElementById("countdown").innerHTML = "EXPIRED";
}
}, 1000);
</script>
</body>
</html>
This example uses a background-image
property to set a background image for the page , and sets the size of the image to cover
so it covers the entire background . It also sets a font family of Arial
and sans-serif
for the text.
You will need to replace “background-image.jpg” with the actual path of your image. If the image is in the same folder as your HTML file, you can simply use the file name (e.g “background-image.jpg”)
You can also customize the font-colors as you like, also you can add
background positioning and repeat propertis to adjust how the image is displayed on the page. You can also add other CSS properties to adjust the layout of the page, such as margins, padding, and dimensions.
You can also experiment with different font colors, you can assign diferent colors to the h1
, p
, and #countdown
elements. You can use either colour names (ex “red”, “blue”, etc ) or HEX codes (ex “#ff0000”, “#0000ff”, etc ) to specify the colors .
additionally you can use google font or other web font to make your coming soon page more attractive
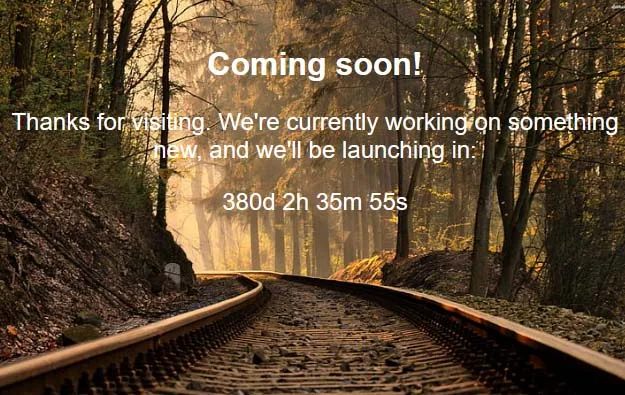